ScatterMessage is a reusable container of multiple in-place data buffers. More...
#include <ScatterMessage.h>
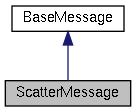
Public Types |
|
enum | { MaxScatterElements = MAX_CLIENT_SCATTER_ELEMENTS } |
enum | Service { Unreliable = UNRELIABLE_MESS, Reliable = RELIABLE_MESS, FIFO = FIFO_MESS, Causal = CAUSAL_MESS, Agreed = AGREED_MESS, Safe = SAFE_MESS, SelfDiscard = SELF_DISCARD, DropReceive = DROP_RECV, UnreliableSelfDiscard = Unreliable | SelfDiscard, ReliableSelfDiscard = Reliable | SelfDiscard, FIFOSelfDiscard = FIFO | SelfDiscard, CausalSelfDiscard = Causal | SelfDiscard, AgreedSelfDiscard = Agreed | SelfDiscard, SafeSelfDiscard = Safe | SelfDiscard } |
Service is not a proper enumeration, but rather a specification of constants corresponding to the Spread service type flags. More... |
|
typedef Spread::int16 | message_type |
Defines the type for 16-bit message type identifiers. More... |
|
typedef Spread::service | service_type |
Defines the type for specifying service types. More... |
|
Public Member Functions |
|
ScatterMessage () | |
Creates a ScatterMessage with a size of zero and no message parts. More... |
|
virtual unsigned int | size () const |
Returns the total size of the message (comprising all of its parts) in bytes. More... |
|
virtual void | clear () |
Clears the message for reuse, resetting both its size and number of message parts to zero. More... |
|
unsigned int | count_message_parts () const |
Returns the number of parts that have been added to the message. More... |
|
unsigned int | count_message_objects () const |
Returns the number of Message instances that have been added to the message. More... |
|
bool | add (const void *data, const unsigned int size) |
Adds a message part to the ScatterMessage with a designated number of bytes indicating either the capacity of the buffer (for receiving) or the length of its contents (for sending). More... |
|
bool | add (const Message &message) |
Adds a Message as a message part. More... |
|
void | set_service (const service_type service) |
Sets the service type of the message. More... |
|
service_type | service () const |
Returns the service type requested (for sends) or sent under (for receives) of the message. More... |
|
void | set_type (const message_type type) |
Sets the message type identifier. More... |
|
message_type | type () const |
Returns the type of the message. More... |
|
void | set_sender (const string &sender) |
Sets the message sender. More... |
|
const string & | sender () const |
Returns the message sender. More... |
|
void | set_endian_mismatch (const bool mismatch=true) |
Sets the endian mismatch flag to the specified value. More... |
|
bool | endian_mismatch () const |
Returns true if there is an endian mismatch between sender and receiver, false otherwise. More... |
|
void | set_agreed () |
Sets the service type to BaseMessage::Agreed. More... |
|
bool | is_agreed () const |
Returns true if service type is BaseMessage::Agreed, false otherwise. More... |
|
void | set_causal () |
Sets the service type to BaseMessage::Causal. More... |
|
bool | is_causal () const |
Returns true if service type is BaseMessage::Causal, false otherwise. More... |
|
void | set_fifo () |
Sets the service type to BaseMessage::FIFO. More... |
|
bool | is_fifo () const |
Returns true if service type is BaseMessage::FIFO, false otherwise. More... |
|
void | set_reliable () |
Sets the service type to BaseMessage::Reliable. More... |
|
bool | is_reliable () const |
Returns true if service type is BaseMessage::Reliable, false otherwise. More... |
|
void | set_unreliable () |
Sets the service type to BaseMessage::Unreliable. More... |
|
bool | is_unreliable () const |
Returns true if service type is BaseMessage::Unreliable, false otherwise. More... |
|
void | set_safe () |
Sets the service type to BaseMessage::Safe. More... |
|
bool | is_safe () const |
Returns true if service type is BaseMessage::Safe, false otherwise. More... |
|
void | set_self_discard (const bool discard=true) |
Adds or removes the BaseMessage::SelfDiscard flag to or from the service type. More... |
|
bool | is_self_discard () const |
Returns true if service type has the BaseMessage::SelfDiscard flag set, false otherwise. More... |
|
bool | is_regular () const |
Returns true if this is a regular data (as opposed to membership) message, false otherwise. More... |
|
bool | is_membership () const |
Returns true if this is a membership message, false otherwise. More... |
|
Protected Attributes |
|
message_type | _type |
service_type | _service_type |
bool | _endian_mismatch |
string | _sender |
Friends |
|
class | Mailbox |
Detailed Description
ScatterMessage is a reusable container of multiple in-place data buffers.
It can be populated with Message instances or straight data pointers. However, the auto-resizing feature of Mailbox::receive will be exercised only if the ScatterMessage contains at least one Message instance. You will want to use a ScatterMessage primarily when you want to avoid multiple buffer copies. For example, if you know the format of an incoming message you can add data pointers for each message part instead of parsing the message and copying the data. Also, instead of allocating a message buffer and copying multiple data items to it for a send, you can populate a ScatterMessage with pointers to the data items and avoid an extra copy.
We do not document ScatterMessage protected members because they are intended only for internal library use.
Definition at line 48 of file ScatterMessage.h.
Member Typedef Documentation
|
inherited |
Defines the type for 16-bit message type identifiers.
Definition at line 63 of file BaseMessage.h.
|
inherited |
Defines the type for specifying service types.
Definition at line 66 of file BaseMessage.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
MaxScatterElements |
The maximum number of elements that can be added to a scatter message. |
Definition at line 51 of file ScatterMessage.h.
|
inherited |
Service is not a proper enumeration, but rather a specification of constants corresponding to the Spread service type flags.
We do not document the meaning of these flags here. See the Spread C API documentation to understand their meaning. We will note, however, that DropReceive is not a service type, but rather a flag instructing the Spread receive functions to truncate messages and group lists if the buffers are too small. There should be no need to use this constant in the API as it is handled by Mailbox::set_drop_receive.
Enumerator | |
---|---|
Unreliable | |
Reliable | |
FIFO | |
Causal | |
Agreed | |
Safe | |
SelfDiscard | |
DropReceive | |
UnreliableSelfDiscard | |
ReliableSelfDiscard | |
FIFOSelfDiscard | |
CausalSelfDiscard | |
AgreedSelfDiscard | |
SafeSelfDiscard |
Definition at line 81 of file BaseMessage.h.
Constructor & Destructor Documentation
|
inline |
Creates a ScatterMessage with a size of zero and no message parts.
See BaseMessage() for the default values of various properties, including service type.
Definition at line 133 of file ScatterMessage.h.
Member Function Documentation
bool ScatterMessage::add | ( | const void * | data, |
const unsigned int | size | ||
) |
Adds a message part to the ScatterMessage with a designated number of bytes indicating either the capacity of the buffer (for receiving) or the length of its contents (for sending).
- Parameters
-
data A pointer to the data buffer to add. size The number of bytes to be filled in to or read from the data buffer.
- Returns
- true if the message part was added, false if there's no more parts can be added.
Definition at line 116 of file ScatterMessage.cc.
References __END_NS_SSRC_SPREAD, MaxScatterElements, and size().
Referenced by add(), Mailbox::add_message_part(), and count_message_objects().
|
inline |
Adds a Message as a message part.
ScatterMessage will store a pointer to the original Message; therefore you should not alter the Message between the time it is added and when the send or receive is performed. Note that for receives, each Message added will be filled up to its capacity, not its size. The size will be ajdusted to reflect the amount of data written to the Message. The capacity will not be increased to accommodate more data except for the last Message added to the ScatterMessage. If you don't want this behavior, use add(const void *, const unsigned int) instead, with &message[0]
as the data argument. When using fixed-length message parts, it is often useful to tack on a Message as the last message part to store any unexpected overflow.
- Parameters
-
message The Message to add.
- Returns
- true if the message part was added, false if no more parts can be added.
Definition at line 197 of file ScatterMessage.h.
References __END_NS_SSRC_SPREAD, add(), and Message::size().
|
inlinevirtual |
Clears the message for reuse, resetting both its size and number of message parts to zero.
Implements BaseMessage.
Definition at line 150 of file ScatterMessage.h.
Referenced by Mailbox::clear_message_parts().
|
inline |
Returns the number of Message instances that have been added to the message.
This number will be different from that returned by count_message_parts() if any straight pointers have ben added.
- Returns
- The number of Message instances that have been added to the message.
Definition at line 172 of file ScatterMessage.h.
References add().
Referenced by if().
|
inline |
Returns the number of parts that have been added to the message.
- Returns
- The number of parts that have been added to the message.
Definition at line 160 of file ScatterMessage.h.
Referenced by Mailbox::count_message_parts().
|
inlineinherited |
Returns true if there is an endian mismatch between sender and receiver, false otherwise.
This only has meaning for received messages.
- Returns
- true if there is an endian mismatch between sender and receiver, false otherwise.
Definition at line 227 of file BaseMessage.h.
References BaseMessage::_endian_mismatch.
|
inlineinherited |
Returns true if service type is BaseMessage::Agreed, false otherwise.
- Returns
- true if service type is BaseMessage::Agreed, false otherwise.
Definition at line 240 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type is BaseMessage::Causal, false otherwise.
- Returns
- true if service type is BaseMessage::Causal, false otherwise.
Definition at line 253 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type is BaseMessage::FIFO, false otherwise.
- Returns
- true if service type is BaseMessage::FIFO, false otherwise.
Definition at line 266 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if this is a membership message, false otherwise.
- Returns
- true if this is a membership message, false otherwise.
Definition at line 345 of file BaseMessage.h.
References __END_NS_SSRC_SPREAD, and BaseMessage::service().
|
inlineinherited |
Returns true if this is a regular data (as opposed to membership) message, false otherwise.
- Returns
- true if this is a regular message, false otherwise.
Definition at line 337 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type is BaseMessage::Reliable, false otherwise.
- Returns
- true if service type is BaseMessage::Reliable, false otherwise.
Definition at line 279 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type is BaseMessage::Safe, false otherwise.
- Returns
- true if service type is BaseMessage::Safe, false otherwise.
Definition at line 305 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type has the BaseMessage::SelfDiscard flag set, false otherwise.
- Returns
- true if service type has the BaseMessage::SelfDiscard flag set, false otherwise.
Definition at line 328 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns true if service type is BaseMessage::Unreliable, false otherwise.
- Returns
- true if service type is BaseMessage::Unreliable, false otherwise.
Definition at line 292 of file BaseMessage.h.
References BaseMessage::service().
|
inlineinherited |
Returns the message sender.
This only has meaning for received messages.
- Returns
- The message sender.
Definition at line 209 of file BaseMessage.h.
References BaseMessage::_sender.
Referenced by BaseMessage::set_sender().
|
inlineinherited |
Returns the service type requested (for sends) or sent under (for receives) of the message.
- Returns
- The service type of the message.
Definition at line 177 of file BaseMessage.h.
References BaseMessage::_service_type.
Referenced by BaseMessage::is_agreed(), BaseMessage::is_causal(), BaseMessage::is_fifo(), BaseMessage::is_membership(), BaseMessage::is_regular(), BaseMessage::is_reliable(), BaseMessage::is_safe(), BaseMessage::is_self_discard(), BaseMessage::is_unreliable(), and BaseMessage::set_service().
|
inlineinherited |
Sets the service type to BaseMessage::Agreed.
Definition at line 232 of file BaseMessage.h.
References BaseMessage::Agreed, and BaseMessage::set_service().
|
inlineinherited |
Sets the service type to BaseMessage::Causal.
Definition at line 245 of file BaseMessage.h.
References BaseMessage::Causal, and BaseMessage::set_service().
|
inlineinherited |
Sets the endian mismatch flag to the specified value.
- Parameters
-
mismatch The mismatch value.
Definition at line 217 of file BaseMessage.h.
Referenced by if().
|
inlineinherited |
Sets the service type to BaseMessage::FIFO.
Definition at line 258 of file BaseMessage.h.
References BaseMessage::FIFO, and BaseMessage::set_service().
|
inlineinherited |
Sets the service type to BaseMessage::Reliable.
Definition at line 271 of file BaseMessage.h.
References BaseMessage::Reliable, and BaseMessage::set_service().
|
inlineinherited |
Sets the service type to BaseMessage::Safe.
Definition at line 297 of file BaseMessage.h.
References BaseMessage::Safe, and BaseMessage::set_service().
|
inlineinherited |
Adds or removes the BaseMessage::SelfDiscard flag to or from the service type.
- Parameters
-
discard true to set the BaseMessage::SelfDiscard flag, false to remove it.
Definition at line 316 of file BaseMessage.h.
References BaseMessage::SelfDiscard.
|
inlineinherited |
Sets the message sender.
- Parameters
-
sender The message sender.
Definition at line 201 of file BaseMessage.h.
References BaseMessage::sender().
Referenced by if().
|
inlineinherited |
Sets the service type of the message.
- Parameters
-
service The service type of the message.
Definition at line 167 of file BaseMessage.h.
References BaseMessage::service().
Referenced by if(), Mailbox::send(), BaseMessage::set_agreed(), BaseMessage::set_causal(), BaseMessage::set_fifo(), BaseMessage::set_reliable(), BaseMessage::set_safe(), and BaseMessage::set_unreliable().
|
inlineinherited |
Sets the message type identifier.
- Parameters
-
type The new message type.
Definition at line 185 of file BaseMessage.h.
References BaseMessage::type().
Referenced by if(), and Mailbox::send().
|
inlineinherited |
Sets the service type to BaseMessage::Unreliable.
Definition at line 284 of file BaseMessage.h.
References BaseMessage::set_service(), and BaseMessage::Unreliable.
|
inlinevirtual |
Returns the total size of the message (comprising all of its parts) in bytes.
- Returns
- The total size of the message in bytes.
Implements BaseMessage.
Definition at line 142 of file ScatterMessage.h.
|
inlineinherited |
Returns the type of the message.
- Returns
- The type of the message.
Definition at line 193 of file BaseMessage.h.
References BaseMessage::_type.
Referenced by BaseMessage::set_type().
Friends And Related Function Documentation
|
friend |
Definition at line 60 of file ScatterMessage.h.
Member Data Documentation
|
protectedinherited |
Definition at line 115 of file BaseMessage.h.
Referenced by BaseMessage::endian_mismatch().
|
protectedinherited |
Definition at line 116 of file BaseMessage.h.
Referenced by BaseMessage::sender().
|
protectedinherited |
Definition at line 114 of file BaseMessage.h.
Referenced by BaseMessage::service().
|
protectedinherited |
Definition at line 113 of file BaseMessage.h.
Referenced by BaseMessage::type().
The documentation for this class was generated from the following files: