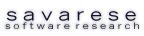
Go to the documentation of this file.
21 #ifndef __SSRC_SPREAD_BASE_MESSAGE_H
22 #define __SSRC_SPREAD_BASE_MESSAGE_H
37 typedef int16 foo_int16;
39 typedef foo_int16 int16;
48 #ifdef LIBSSRCSPREAD_ENABLE_MEMBERSHIP_INFO
90 #define SERVICE_TYPE_DISCARD(s) s ## SelfDiscard = s | SelfDiscard
99 #undef SERVICE_TYPE_DISCARD
104 #ifdef LIBSSRCSPREAD_ENABLE_MEMBERSHIP_INFO
106 void get_vs_set_members(
const Spread::vs_set_info *vs_set,
107 GroupList *members,
unsigned int offset = 0)
const;
124 _type(0), _service_type(
Safe), _endian_mismatch(false), _sender(
"")
127 #ifdef LIBSSRCSPREAD_ENABLE_MEMBERSHIP_INFO
129 virtual int sp_get_membership_info(Spread::membership_info *info)
const = 0;
131 virtual int sp_get_vs_set_members(
const Spread::vs_set_info *vs_set,
133 unsigned int member_names_count)
const = 0;
135 virtual int sp_get_vs_sets_info(Spread::vs_set_info *vs_sets,
136 unsigned int num_vs_sets,
137 unsigned int *index)
const = 0;
151 virtual unsigned int size()
const = 0;
154 virtual void clear() = 0;
156 #ifdef LIBSSRCSPREAD_ENABLE_MEMBERSHIP_INFO
158 void get_membership_info(MembershipInfo & info)
const SSRC_DECL_THROW(
Error);
218 _endian_mismatch = mismatch;
241 return Is_agreed_mess(
service());
254 return Is_causal_mess(
service());
267 return Is_fifo_mess(
service());
280 return Is_reliable_mess(
service());
293 return Is_unreliable_mess(
service());
306 return Is_safe_mess(
service());
329 return Is_self_discard(
service());
338 return Is_regular_mess(
service());
346 return Is_membership_mess(
service());
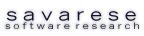
Copyright © 2006-2015 Savarese Software Research Corporation. All rights reserved.
Copyright © 2017 Savarese Software Research Corporation. All rights reserved