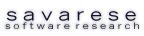
Go to the documentation of this file.
28 void ScatterMessage::init_pre_receive() {
29 int size = _messages.size();
31 for(
int i = 0; i <
size; ++i) {
32 value_type & value = _messages[i];
35 if(value.second >= _scatter.num_elements)
39 m->resize(m->capacity());
41 _scatter.elements[value.second].len = m->
size();
55 void ScatterMessage::init_post_receive(
int bytes_received) {
56 int index = 0, i, len;
57 int size = _messages.size();
60 if(bytes_received > 0)
61 _size = bytes_received;
65 for(i = 0; i < size && bytes_received > 0; ++i, ++index) {
66 value_type & value = _messages[i];
67 int mindex = value.second;
69 if(mindex >= _scatter.num_elements)
73 bytes_received-=_scatter.elements[index++].len;
75 if(bytes_received <= 0)
78 len = _scatter.elements[mindex].len;
86 if(len <= bytes_received)
89 m->resize(bytes_received);
95 for(; i <
size; ++i) {
96 m = _messages[i].first;
117 int i = _scatter.num_elements;
122 ++_scatter.num_elements;
123 _scatter.elements[i].buf =
124 const_cast<char *
>(
static_cast<const char *
>(data));
125 _scatter.elements[i].len =
size;
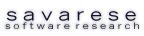
Copyright © 2006-2015 Savarese Software Research Corporation. All rights reserved.
Copyright © 2017 Savarese Software Research Corporation. All rights reserved