#include <Properties.h>
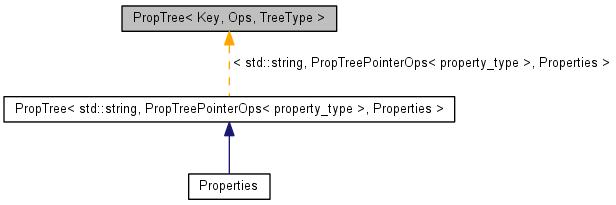
Public Types | |
typedef Key | key_type |
typedef TreeType | tree_type |
typedef Ops | operations |
typedef operations::value_type | value_type |
typedef operations::storage_type | storage_type |
typedef boost::ptr_map < key_type, tree_type > | child_container |
typedef child_container::iterator | child_container_iterator |
typedef child_container::const_iterator | child_container_const_iterator |
typedef child_container::size_type | size_type |
typedef std::pair < child_container_iterator, bool > | insert_result_type |
Public Member Functions | |
PropTree () | |
PropTree (const value_type &v) | |
~PropTree () | |
void | clear () |
bool | is_leaf () const |
const storage_type & | value () const |
storage_type & | value () |
void | set_value (const value_type &v) |
child_container_const_iterator | child_begin () const |
child_container_const_iterator | child_end () const |
tree_type * | find (const key_type &key) |
template<typename... P> | |
tree_type * | find (const key_type &key, const P &...p) |
const tree_type * | find (const key_type &key) const |
template<typename... P> | |
const tree_type * | find (const key_type &key, const P &...p) const |
tree_type * | set (const value_type &val, const key_type &key) |
template<typename... P> | |
tree_type * | set (const value_type &val, const key_type &key, const P &...p) |
tree_type * | create_node (const key_type &key) |
template<typename... P> | |
tree_type * | create_node (const key_type &key, const P &...p) |
size_type | erase (const key_type &key) |
template<typename... P> | |
size_type | erase (const key_type &key, const P &...p) |
template<typename Visitor > | |
void | visit (const Visitor &v) |
template<typename Visitor > | |
void | visit (const Visitor &v) const |
bool | operator== (const tree_type &p) const |
void | merge (tree_type &&p) |
void | merge (const tree_type &p) |
tree_type * | va_set (const value_type &val, const std::va_list &ap) |
tree_type * | va_list_find (const key_type *key, const std::va_list &ap) |
tree_type * | va_find (const key_type *key,...) |
template<typename Iterator > | |
tree_type * | iterator_set (const value_type &val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
size_type | iterator_erase (const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
tree_type * | iterator_find (const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
const tree_type * | iterator_find (const Iterator &begin, const Iterator &end) const |
template<class Archive > | |
void | save (Archive &ar, const unsigned int) const |
template<class Archive > | |
void | load (Archive &ar, const unsigned int) |
Protected Attributes | |
storage_type | _value |
child_container | _children |
Static Protected Attributes | |
static const operations | Value = typename PropTree<K, T, Tr>::operations() |
Detailed Description
template<typename Key, typename Ops, typename TreeType>
class PropTree< Key, Ops, TreeType >
Definition at line 127 of file utility/Properties.h.
Member Typedef Documentation
typedef boost::ptr_map<key_type, tree_type> PropTree< Key, Ops, TreeType >::child_container |
Definition at line 135 of file utility/Properties.h.
typedef child_container::const_iterator PropTree< Key, Ops, TreeType >::child_container_const_iterator |
Definition at line 137 of file utility/Properties.h.
typedef child_container::iterator PropTree< Key, Ops, TreeType >::child_container_iterator |
Definition at line 136 of file utility/Properties.h.
typedef std::pair<child_container_iterator, bool> PropTree< Key, Ops, TreeType >::insert_result_type |
Definition at line 139 of file utility/Properties.h.
typedef Key PropTree< Key, Ops, TreeType >::key_type |
Definition at line 129 of file utility/Properties.h.
typedef Ops PropTree< Key, Ops, TreeType >::operations |
Definition at line 131 of file utility/Properties.h.
typedef child_container::size_type PropTree< Key, Ops, TreeType >::size_type |
Definition at line 138 of file utility/Properties.h.
typedef operations::storage_type PropTree< Key, Ops, TreeType >::storage_type |
Definition at line 133 of file utility/Properties.h.
typedef TreeType PropTree< Key, Ops, TreeType >::tree_type |
Definition at line 130 of file utility/Properties.h.
typedef operations::value_type PropTree< Key, Ops, TreeType >::value_type |
Definition at line 132 of file utility/Properties.h.
Constructor & Destructor Documentation
PropTree< Key, Ops, TreeType >::PropTree | ( | ) | [inline] |
Definition at line 150 of file utility/Properties.h.
PropTree< Key, Ops, TreeType >::PropTree | ( | const value_type & | v | ) | [inline, explicit] |
Definition at line 152 of file utility/Properties.h.
PropTree< Key, Ops, TreeType >::~PropTree | ( | ) | [inline] |
Definition at line 155 of file utility/Properties.h.
Member Function Documentation
child_container_const_iterator PropTree< Key, Ops, TreeType >::child_begin | ( | ) | const [inline] |
Definition at line 179 of file utility/Properties.h.
child_container_const_iterator PropTree< Key, Ops, TreeType >::child_end | ( | ) | const [inline] |
Definition at line 183 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::clear | ( | ) | [inline] |
Definition at line 159 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::create_node | ( | const key_type & | key | ) | [inline] |
Definition at line 247 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::create_node(), and ServiceProtocolProcessor::get_status().
tree_type* PropTree< Key, Ops, TreeType >::create_node | ( | const key_type & | key, |
const P &... | p | ||
) | [inline] |
Definition at line 261 of file utility/Properties.h.
size_type PropTree< Key, Ops, TreeType >::erase | ( | const key_type & | key | ) | [inline] |
Definition at line 273 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::erase(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_erase().
size_type PropTree< Key, Ops, TreeType >::erase | ( | const key_type & | key, |
const P &... | p | ||
) | [inline] |
Definition at line 278 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::find | ( | const key_type & | key | ) | [inline] |
Definition at line 187 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::create_node(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::erase(), get_number(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_erase(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_find(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_list_find(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_set().
tree_type* PropTree< Key, Ops, TreeType >::find | ( | const key_type & | key, |
const P &... | p | ||
) | [inline] |
Definition at line 195 of file utility/Properties.h.
const tree_type* PropTree< Key, Ops, TreeType >::find | ( | const key_type & | key | ) | const [inline] |
Definition at line 203 of file utility/Properties.h.
const tree_type* PropTree< Key, Ops, TreeType >::find | ( | const key_type & | key, |
const P &... | p | ||
) | const [inline] |
Definition at line 211 of file utility/Properties.h.
bool PropTree< Key, Ops, TreeType >::is_leaf | ( | ) | const [inline] |
Definition at line 163 of file utility/Properties.h.
Referenced by PropertiesToString::enter(), PropertiesToString::leave(), PropertiesToString::operator()(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::visit().
size_type PropTree< Key, Ops, TreeType >::iterator_erase | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | [inline] |
Definition at line 465 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::iterator_find | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | [inline] |
Definition at line 488 of file utility/Properties.h.
const tree_type* PropTree< Key, Ops, TreeType >::iterator_find | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | const [inline] |
Definition at line 502 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::iterator_set | ( | const value_type & | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Reimplemented in Properties.
Definition at line 444 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::load | ( | Archive & | ar, |
const unsigned | int | ||
) | [inline] |
Definition at line 521 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::merge | ( | tree_type && | p | ) | [inline] |
Definition at line 340 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::merge | ( | const tree_type & | p | ) | [inline] |
Definition at line 369 of file utility/Properties.h.
bool PropTree< Key, Ops, TreeType >::operator== | ( | const tree_type & | p | ) | const [inline] |
Definition at line 319 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::save | ( | Archive & | ar, |
const unsigned | int | ||
) | const [inline] |
Definition at line 516 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::set | ( | const value_type & | val, |
const key_type & | key | ||
) | [inline] |
Definition at line 219 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::set | ( | const value_type & | val, |
const key_type & | key, | ||
const P &... | p | ||
) | [inline] |
Definition at line 235 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::set_value | ( | const value_type & | v | ) | [inline] |
Definition at line 175 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_set().
tree_type* PropTree< Key, Ops, TreeType >::va_find | ( | const key_type * | key, |
... | |||
) | [inline] |
Definition at line 429 of file utility/Properties.h.
tree_type* PropTree< Key, Ops, TreeType >::va_list_find | ( | const key_type * | key, |
const std::va_list & | ap | ||
) | [inline] |
Definition at line 417 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_find().
tree_type* PropTree< Key, Ops, TreeType >::va_set | ( | const value_type & | val, |
const std::va_list & | ap | ||
) | [inline] |
Definition at line 398 of file utility/Properties.h.
const storage_type& PropTree< Key, Ops, TreeType >::value | ( | ) | const [inline] |
Definition at line 167 of file utility/Properties.h.
Referenced by PropertiesToString::enter(), get_number(), and PropertiesToString::operator()().
storage_type& PropTree< Key, Ops, TreeType >::value | ( | ) | [inline] |
Definition at line 171 of file utility/Properties.h.
void PropTree< Key, Ops, TreeType >::visit | ( | const Visitor & | v | ) | [inline] |
Definition at line 290 of file utility/Properties.h.
Referenced by ServiceProtocolProcessor::get_status(), PropertiesToString::operator()(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::visit().
void PropTree< Key, Ops, TreeType >::visit | ( | const Visitor & | v | ) | const [inline] |
Definition at line 305 of file utility/Properties.h.
Member Data Documentation
child_container PropTree< Key, Ops, TreeType >::_children [protected] |
Definition at line 146 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::child_begin(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::child_end(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::clear(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::create_node(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::erase(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::find(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::is_leaf(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::load(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::merge(), Properties::operator=(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::operator==(), Properties::Properties(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::save(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_set(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::visit().
storage_type PropTree< Key, Ops, TreeType >::_value [protected] |
Definition at line 145 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::load(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::merge(), Properties::operator=(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::operator==(), Properties::Properties(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::save(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::value(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::~PropTree().
PropTree< K, T, Tr >::operations const PropTree< K, T, Tr >::Value = typename PropTree<K, T, Tr>::operations() [static, protected] |
Definition at line 143 of file utility/Properties.h.
Referenced by PropTree< std::string, PropTreePointerOps< property_type >, Properties >::load(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::merge(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::operator==(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::~PropTree().
The documentation for this class was generated from the following file: