#include <Properties.h>
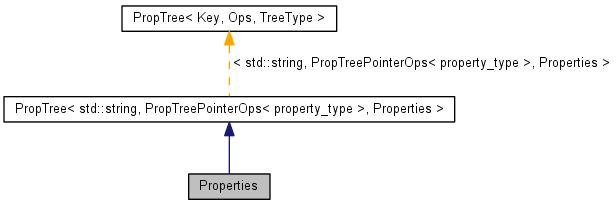
Public Types | |
typedef PropTree< std::string, PropTreePointerOps < property_type >, Properties > | super |
typedef std::string | key_type |
typedef Properties | tree_type |
typedef PropTreePointerOps < property_type > | operations |
typedef operations::value_type | value_type |
typedef operations::storage_type | storage_type |
typedef boost::ptr_map < key_type, tree_type > | child_container |
typedef child_container::iterator | child_container_iterator |
typedef child_container::const_iterator | child_container_const_iterator |
typedef child_container::size_type | size_type |
typedef std::pair < child_container_iterator, bool > | insert_result_type |
Public Member Functions | |
Properties () | |
Properties (const value_type &v) | |
Properties (const Properties &p) | |
Properties & | operator= (const Properties &p) |
Properties (Properties &&p) | |
Properties & | operator= (Properties &&p) |
bool | is_null () const |
template<typename... P> | |
Properties * | set (const char *const val, const P &...p) |
template<typename... P> | |
Properties * | set (const long val, const P &...p) |
template<typename... P> | |
Properties * | set (const unsigned long val, const P &...p) |
template<typename... P> | |
Properties * | set (const char val, const P &...p) |
template<typename... P> | |
Properties * | set (const signed char val, const P &...p) |
template<typename... P> | |
Properties * | set (const unsigned char val, const P &...p) |
template<typename... P> | |
Properties * | set (const bool val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::int32_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::uint32_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::int16_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::uint16_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::int64_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const std::uint64_t val, const P &...p) |
template<typename... P> | |
Properties * | set (const float val, const P &...p) |
template<typename... P> | |
Properties * | set (const double val, const P &...p) |
template<typename... P> | |
Properties * | set (const value_type &val, const P &...p) |
template<typename Iterator > | |
Properties * | iterator_set (const char *const val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const long val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const unsigned long val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const char val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const signed char val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const unsigned char val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const bool val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const std::int32_t val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const std::uint32_t val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const std::int64_t val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const std::uint64_t val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const float val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const double val, const Iterator &begin, const Iterator &end) |
template<typename Iterator > | |
Properties * | iterator_set (const value_type &val, const Iterator &begin, const Iterator &end) |
template<typename T > | |
T * | get_ptr () const |
template<typename T , typename... P> | |
T * | get_ptr (const P &...p) const |
property_vector & | create_property_vector () |
template<typename... P> | |
property_vector & | create_property_vector (const P &...p) |
primitive_property_vector & | create_primitive_property_vector () |
template<typename... P> | |
primitive_property_vector & | create_primitive_property_vector (const P &...p) |
template<typename T > | |
const T & | get (const T &default_value=T()) const |
template<typename T , typename... P> | |
const T & | get (const T &default_value, const P &...p) const |
template<typename T > | |
void | va_set (const T &val,...) |
template<class Archive > | |
void | serialize (Archive &ar, const unsigned int) |
void | clear () |
bool | is_leaf () const |
const storage_type & | value () const |
storage_type & | value () |
void | set_value (const value_type &v) |
child_container_const_iterator | child_begin () const |
child_container_const_iterator | child_end () const |
tree_type * | find (const key_type &key) |
tree_type * | find (const key_type &key, const P &...p) |
const tree_type * | find (const key_type &key) const |
const tree_type * | find (const key_type &key, const P &...p) const |
tree_type * | set (const value_type &val, const key_type &key) |
tree_type * | set (const value_type &val, const key_type &key, const P &...p) |
tree_type * | create_node (const key_type &key) |
tree_type * | create_node (const key_type &key, const P &...p) |
size_type | erase (const key_type &key) |
size_type | erase (const key_type &key, const P &...p) |
void | visit (const Visitor &v) |
void | visit (const Visitor &v) const |
bool | operator== (const tree_type &p) const |
void | merge (tree_type &&p) |
void | merge (const tree_type &p) |
tree_type * | va_set (const value_type &val, const std::va_list &ap) |
tree_type * | va_list_find (const key_type *key, const std::va_list &ap) |
tree_type * | va_find (const key_type *key,...) |
size_type | iterator_erase (const Iterator &begin, const Iterator &end) |
tree_type * | iterator_find (const Iterator &begin, const Iterator &end) |
const tree_type * | iterator_find (const Iterator &begin, const Iterator &end) const |
void | save (Archive &ar, const unsigned int) const |
void | load (Archive &ar, const unsigned int) |
Protected Attributes | |
storage_type | _value |
child_container | _children |
Static Protected Attributes | |
static const operations | Value |
Detailed Description
Definition at line 578 of file utility/Properties.h.
Member Typedef Documentation
typedef boost::ptr_map<key_type, tree_type> PropTree< std::string , PropTreePointerOps< property_type > , Properties >::child_container [inherited] |
Definition at line 135 of file utility/Properties.h.
typedef child_container::const_iterator PropTree< std::string , PropTreePointerOps< property_type > , Properties >::child_container_const_iterator [inherited] |
Definition at line 137 of file utility/Properties.h.
typedef child_container::iterator PropTree< std::string , PropTreePointerOps< property_type > , Properties >::child_container_iterator [inherited] |
Definition at line 136 of file utility/Properties.h.
typedef std::pair<child_container_iterator, bool> PropTree< std::string , PropTreePointerOps< property_type > , Properties >::insert_result_type [inherited] |
Definition at line 139 of file utility/Properties.h.
typedef std::string PropTree< std::string , PropTreePointerOps< property_type > , Properties >::key_type [inherited] |
Definition at line 129 of file utility/Properties.h.
typedef PropTreePointerOps< property_type > PropTree< std::string , PropTreePointerOps< property_type > , Properties >::operations [inherited] |
Definition at line 131 of file utility/Properties.h.
typedef child_container::size_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::size_type [inherited] |
Definition at line 138 of file utility/Properties.h.
typedef operations::storage_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::storage_type [inherited] |
Definition at line 133 of file utility/Properties.h.
Definition at line 584 of file utility/Properties.h.
typedef Properties PropTree< std::string , PropTreePointerOps< property_type > , Properties >::tree_type [inherited] |
Definition at line 130 of file utility/Properties.h.
typedef operations::value_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::value_type [inherited] |
Definition at line 132 of file utility/Properties.h.
Constructor & Destructor Documentation
Properties::Properties | ( | ) | [inline] |
Definition at line 586 of file utility/Properties.h.
Properties::Properties | ( | const value_type & | v | ) | [inline, explicit] |
Definition at line 588 of file utility/Properties.h.
Properties::Properties | ( | const Properties & | p | ) | [inline] |
Definition at line 590 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_children, PropTree< Key, Ops, TreeType >::_children, PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value, PropTree< Key, Ops, TreeType >::_value, PropTreePointerOps< T >::free(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::Value.
Properties::Properties | ( | Properties && | p | ) | [inline, explicit] |
Definition at line 618 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_children, and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value.
Member Function Documentation
child_container_const_iterator PropTree< std::string , PropTreePointerOps< property_type > , Properties >::child_begin | ( | ) | const [inline, inherited] |
Definition at line 179 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
child_container_const_iterator PropTree< std::string , PropTreePointerOps< property_type > , Properties >::child_end | ( | ) | const [inline, inherited] |
Definition at line 183 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::clear | ( | ) | [inline, inherited] |
Definition at line 159 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::create_node | ( | const key_type & | key | ) | [inline, inherited] |
Definition at line 247 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, and PropTree< Key, Ops, TreeType >::find().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::create_node | ( | const key_type & | key, |
const P &... | p | ||
) | [inline, inherited] |
Definition at line 261 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::create_node(), and PropTree< Key, Ops, TreeType >::find().
Definition at line 868 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value().
Referenced by ServiceProtocolProcessor::get_status().
primitive_property_vector& Properties::create_primitive_property_vector | ( | const P &... | p | ) | [inline] |
Definition at line 874 of file utility/Properties.h.
property_vector& Properties::create_property_vector | ( | ) | [inline] |
Definition at line 858 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value().
property_vector& Properties::create_property_vector | ( | const P &... | p | ) | [inline] |
Definition at line 864 of file utility/Properties.h.
size_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::erase | ( | const key_type & | key | ) | [inline, inherited] |
Definition at line 273 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
size_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::erase | ( | const key_type & | key, |
const P &... | p | ||
) | [inline, inherited] |
Definition at line 278 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::erase(), and PropTree< Key, Ops, TreeType >::find().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::find | ( | const key_type & | key | ) | [inline, inherited] |
Definition at line 187 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
Referenced by get_ptr().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::find | ( | const key_type & | key, |
const P &... | p | ||
) | [inline, inherited] |
Definition at line 195 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
const tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::find | ( | const key_type & | key | ) | const [inline, inherited] |
Definition at line 203 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
const tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::find | ( | const key_type & | key, |
const P &... | p | ||
) | const [inline, inherited] |
Definition at line 211 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
const T& Properties::get | ( | const T & | default_value = T() | ) | const [inline] |
Definition at line 879 of file utility/Properties.h.
const T& Properties::get | ( | const T & | default_value, |
const P &... | p | ||
) | const [inline] |
Definition at line 890 of file utility/Properties.h.
T* Properties::get_ptr | ( | ) | const [inline] |
Definition at line 838 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value.
Referenced by get_ptr().
T* Properties::get_ptr | ( | const P &... | p | ) | const [inline] |
Definition at line 849 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::find(), and get_ptr().
bool PropTree< std::string , PropTreePointerOps< property_type > , Properties >::is_leaf | ( | ) | const [inline, inherited] |
Definition at line 163 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children.
bool Properties::is_null | ( | ) | const [inline] |
Definition at line 633 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value.
Referenced by get_number().
size_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::iterator_erase | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | [inline, inherited] |
Definition at line 465 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::erase(), and PropTree< Key, Ops, TreeType >::find().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::iterator_find | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | [inline, inherited] |
Definition at line 488 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::find().
const tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::iterator_find | ( | const Iterator & | begin, |
const Iterator & | end | ||
) | const [inline, inherited] |
Definition at line 502 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::find().
Properties* Properties::iterator_set | ( | const char *const | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 724 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set(), and string.
Properties* Properties::iterator_set | ( | const long | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 733 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const unsigned long | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 741 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const char | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 750 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const signed char | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 758 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const unsigned char | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 766 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const bool | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 774 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const std::int32_t | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 782 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const std::uint32_t | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 790 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const std::int64_t | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 798 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const std::uint64_t | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 806 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const float | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 814 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const double | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Definition at line 822 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
Properties* Properties::iterator_set | ( | const value_type & | val, |
const Iterator & | begin, | ||
const Iterator & | end | ||
) | [inline] |
Reimplemented from PropTree< std::string, PropTreePointerOps< property_type >, Properties >.
Definition at line 830 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::iterator_set().
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::load | ( | Archive & | ar, |
const unsigned | int | ||
) | [inline, inherited] |
Definition at line 521 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::_value, and PropTree< Key, Ops, TreeType >::Value.
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::merge | ( | tree_type && | p | ) | [inline, inherited] |
Definition at line 340 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::_value, and PropTree< Key, Ops, TreeType >::Value.
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::merge | ( | const tree_type & | p | ) | [inline, inherited] |
Definition at line 369 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::_value, and PropTree< Key, Ops, TreeType >::Value.
Properties& Properties::operator= | ( | const Properties & | p | ) | [inline] |
Definition at line 603 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_children, PropTree< Key, Ops, TreeType >::_children, PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value, PropTree< Key, Ops, TreeType >::_value, PropTreePointerOps< T >::free(), PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set_value(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::Value.
Properties& Properties::operator= | ( | Properties && | p | ) | [inline] |
Definition at line 624 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_children, PropTree< std::string, PropTreePointerOps< property_type >, Properties >::_value, PropTreePointerOps< T >::free(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::Value.
bool PropTree< std::string , PropTreePointerOps< property_type > , Properties >::operator== | ( | const tree_type & | p | ) | const [inline, inherited] |
Definition at line 319 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::_value, and PropTree< Key, Ops, TreeType >::Value.
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::save | ( | Archive & | ar, |
const unsigned | int | ||
) | const [inline, inherited] |
Definition at line 516 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, and PropTree< Key, Ops, TreeType >::_value.
void Properties::serialize | ( | Archive & | ar, |
const unsigned | int | ||
) | [inline] |
Definition at line 914 of file utility/Properties.h.
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::set | ( | const value_type & | val, |
const key_type & | key | ||
) | [inline, inherited] |
Definition at line 219 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::find(), and PropTree< Key, Ops, TreeType >::set_value().
Referenced by set().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::set | ( | const value_type & | val, |
const key_type & | key, | ||
const P &... | p | ||
) | [inline, inherited] |
Definition at line 235 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::find(), and set().
Properties* Properties::set | ( | const char *const | val, |
const P &... | p | ||
) | [inline] |
Definition at line 640 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set(), and string.
Referenced by bind_group_session_properties(), ServiceProtocolProcessor::get_status(), and PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const long | val, |
const P &... | p | ||
) | [inline] |
Definition at line 647 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const unsigned long | val, |
const P &... | p | ||
) | [inline] |
Definition at line 652 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const char | val, |
const P &... | p | ||
) | [inline] |
Definition at line 658 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const signed char | val, |
const P &... | p | ||
) | [inline] |
Definition at line 663 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const unsigned char | val, |
const P &... | p | ||
) | [inline] |
Definition at line 668 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const bool | val, |
const P &... | p | ||
) | [inline] |
Definition at line 673 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::int32_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 678 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::uint32_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 683 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::int16_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 688 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::uint16_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 693 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::int64_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 698 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const std::uint64_t | val, |
const P &... | p | ||
) | [inline] |
Definition at line 703 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const float | val, |
const P &... | p | ||
) | [inline] |
Definition at line 708 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const double | val, |
const P &... | p | ||
) | [inline] |
Definition at line 713 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
Properties* Properties::set | ( | const value_type & | val, |
const P &... | p | ||
) | [inline] |
Definition at line 718 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::set().
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::set_value | ( | const value_type & | v | ) | [inline, inherited] |
Definition at line 175 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_value, and PropTree< Key, Ops, TreeType >::Value.
Referenced by create_primitive_property_vector(), create_property_vector(), operator=(), and Properties().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::va_find | ( | const key_type * | key, |
... | |||
) | [inline, inherited] |
Definition at line 429 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::va_list_find().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::va_list_find | ( | const key_type * | key, |
const std::va_list & | ap | ||
) | [inline, inherited] |
Definition at line 417 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::find().
tree_type* PropTree< std::string , PropTreePointerOps< property_type > , Properties >::va_set | ( | const value_type & | val, |
const std::va_list & | ap | ||
) | [inline, inherited] |
Definition at line 398 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::find(), and PropTree< Key, Ops, TreeType >::set_value().
Referenced by va_set().
void Properties::va_set | ( | const T & | val, |
... | |||
) | [inline] |
Definition at line 903 of file utility/Properties.h.
References PropTree< std::string, PropTreePointerOps< property_type >, Properties >::va_set().
const storage_type& PropTree< std::string , PropTreePointerOps< property_type > , Properties >::value | ( | ) | const [inline, inherited] |
Definition at line 167 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_value.
storage_type& PropTree< std::string , PropTreePointerOps< property_type > , Properties >::value | ( | ) | [inline, inherited] |
Definition at line 171 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_value.
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::visit | ( | const Visitor & | v | ) | [inline, inherited] |
Definition at line 290 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::is_leaf(), and PropTree< Key, Ops, TreeType >::visit().
void PropTree< std::string , PropTreePointerOps< property_type > , Properties >::visit | ( | const Visitor & | v | ) | const [inline, inherited] |
Definition at line 305 of file utility/Properties.h.
References PropTree< Key, Ops, TreeType >::_children, PropTree< Key, Ops, TreeType >::is_leaf(), and PropTree< Key, Ops, TreeType >::visit().
Member Data Documentation
child_container PropTree< std::string , PropTreePointerOps< property_type > , Properties >::_children [protected, inherited] |
Definition at line 146 of file utility/Properties.h.
Referenced by operator=(), and Properties().
storage_type PropTree< std::string , PropTreePointerOps< property_type > , Properties >::_value [protected, inherited] |
Definition at line 145 of file utility/Properties.h.
Referenced by get_ptr(), is_null(), operator=(), and Properties().
const operations PropTree< std::string , PropTreePointerOps< property_type > , Properties >::Value [static, protected, inherited] |
Definition at line 143 of file utility/Properties.h.
Referenced by operator=(), and Properties().
The documentation for this class was generated from the following file: