GroupSessionService is not a standalone service (although it was originally). More...
#include <service.h>
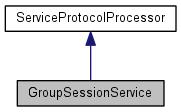
Public Member Functions | |
GroupSessionService (super::caller_type &caller, const wisp_message_protocol protocol, GroupSessionDatabase *db, const GroupSessionInitializer &initializer) SSRC_DECL_THROW(DatabaseException | |
The db argument should be the result of a direct new allocation because GroupSessionService stores it in a scoped_ptr. | |
virtual | ~GroupSessionService () |
virtual unsigned int | check_for_expirations (const sec_type now) |
Protected Types | |
typedef GroupSessionProtocol | protocol_traits |
Protected Member Functions | |
WISP_IMPORT (protocol_traits, MessageCreateGroupSession) | |
WISP_IMPORT (protocol_traits, MessageEndGroupSession) | |
WISP_IMPORT (protocol_traits, MessageExpireGroupSession) | |
WISP_IMPORT (protocol_traits, MessageRelayEvent) | |
WISP_IMPORT (protocol_traits, MessageRelayEventSelfDiscard) | |
WISP_IMPORT (protocol_traits, MessageRelayEvents) | |
WISP_IMPORT (protocol_traits, MessageRelayEventsSelfDiscard) | |
WISP_IMPORT (protocol_traits, MessageFindGroupSessions) | |
WISP_IMPORT (protocol_traits, MessageFindGroupSessionsResult) | |
WISP_IMPORT (protocol_traits, MessageFindGroupSessionsForMember) | |
WISP_IMPORT (protocol_traits, MessageReturnGroupSessionsForMember) | |
WISP_IMPORT (protocol_traits, MessageFindGroupSessionsByType) | |
WISP_IMPORT (protocol_traits, MessageReturnGroupSessionsByType) | |
WISP_IMPORT (protocol_traits, MessageCreateReservation) | |
WISP_IMPORT (protocol_traits, MessageCancelReservation) | |
WISP_IMPORT (protocol_traits, MessageFindMembers) | |
WISP_IMPORT (protocol_traits, MessageFindMembersResult) | |
WISP_IMPORT (protocol_traits, MessageAddMembers) | |
WISP_IMPORT (protocol_traits, MessageRemoveMembers) | |
WISP_IMPORT (protocol_traits, MessageAddMember) | |
WISP_IMPORT (protocol_traits, MessageRemoveMember) | |
WISP_IMPORT (SessionProtocol, MessageExpireSession) | |
WISP_IMPORT (SessionProtocol, MessageLoginSession) | |
WISP_IMPORT (protocol_traits, CallStartGroupSession) | |
WISP_IMPORT (protocol_traits, CallExpireGroupSession) | |
WISP_IMPORT (protocol_traits, CallReturnGroupSessionsForMember) | |
WISP_IMPORT (protocol_traits, CallReturnGroupSessionsByType) | |
WISP_IMPORT (protocol_traits, CallFindGroupSessionsResult) | |
WISP_IMPORT (protocol_traits, CallFindMembersResult) | |
WISP_IMPORT (protocol_traits, CallAddMemberConfirm) | |
WISP_IMPORT (protocol_traits, CallRemoveMemberConfirm) | |
gsid_type | generate_gsid () |
void | recount_sessions () SSRC_DECL_THROW(DatabaseException) |
virtual void | start_group_session (const GroupSession &session, ByteBuffer &payload) |
void | process_request (MessageCreateGroupSession &msg, const MessageInfo &) |
virtual void | end_group_session (const gsid_type gsid) |
void | process_request (const MessageEndGroupSession &msg, const MessageInfo &) |
virtual bool | insert_reservation (const Reservation &reservation, ByteBuffer &payload) SSRC_DECL_THROW(DatabaseException) |
void | process_request (MessageCreateReservation &msg, const MessageInfo &) |
virtual void | confirm_reservation_activation (const GroupSession &session) |
template<typename reservation_type > | |
void | activate_reservation (const reservation_type &reservation, const string &session_name, const member_container &additional_participants) |
void | process_request (const MessageFindGroupSessionsByType &msg, const MessageInfo &msginfo) |
void | process_request (const MessageFindGroupSessions &msg, const MessageInfo &msginfo) |
void | process_request (const MessageFindMembers &msg, const MessageInfo &msginfo) |
void | process_request (const MessageAddMembers &msg, const MessageInfo &) |
void | process_request (const MessageRemoveMembers &msg, const MessageInfo &) |
void | process_request (const MessageAddMember &msg, const MessageInfo &msginfo) |
void | process_request (const MessageRemoveMember &msg, const MessageInfo &msginfo) |
virtual unsigned int | cancel_reservation (const gsid_type gsid, const uid_type requestor, const MessageInfo &) |
void | process_request (const MessageCancelReservation &msg, const MessageInfo &msginfo) |
void | process_request (const MessageFindGroupSessionsForMember &msg, const MessageInfo &msginfo) |
void | process_request (const MessageRelayEvent &msg, const MessageInfo &) |
void | process_request (const MessageRelayEventSelfDiscard &msg, const MessageInfo &) |
void | process_request (MessageRelayEvents &msg, const MessageInfo &) |
void | process_request (MessageRelayEventsSelfDiscard &msg, const MessageInfo &) |
void | process_request (const MessageExpireSession &msg, const MessageInfo &) |
void | process_request (const MessageLoginSession &msg, const MessageInfo &) |
virtual void | transition (State state) |
Protected Attributes | |
const unsigned int | _protocol_id |
const unsigned int | _partition_id |
const unsigned int | _num_partitions |
const unsigned int | _ids_per_expiration_message |
boost::scoped_ptr < GroupSessionDatabase > | _database |
const gsid_type | _gsid_min |
const gsid_type | _gsid_max |
Random< gsid_type > | _random |
TimeValue | _gs_poll_interval |
timeout_ptr | _gs_poll_timeout |
unsigned int | _session_count |
unsigned int | _reservation_count |
const std::string | _gs_name |
Static Protected Attributes | |
static const string | EventGroupExpireGroupSession |
Friends | |
class | NS_SSRC_WISP_SERVICE::ServiceProtocolProcessor< packing_traits > |
Detailed Description
GroupSessionService is not a standalone service (although it was originally).
For scalability reasons, applications that require group sessions should create a custom group session controller that subclasses GroupSessionService. It's not a straightforward procedure, detailed instructions will have to be written.
Definition at line 50 of file group_session/service.h.
Member Typedef Documentation
typedef GroupSessionProtocol GroupSessionService::protocol_traits [protected] |
Definition at line 55 of file group_session/service.h.
Constructor & Destructor Documentation
GroupSessionService::GroupSessionService | ( | super::caller_type & | caller, |
const wisp_message_protocol | protocol, | ||
GroupSessionDatabase * | db, | ||
const GroupSessionInitializer & | initializer | ||
) |
The db argument should be the result of a direct new allocation because GroupSessionService stores it in a scoped_ptr.
This is a kluge to avoid making GroupSessionService a template class.
virtual GroupSessionService::~GroupSessionService | ( | ) | [inline, virtual] |
Definition at line 261 of file group_session/service.h.
Member Function Documentation
void GroupSessionService::activate_reservation | ( | const reservation_type & | reservation, |
const string & | session_name, | ||
const member_container & | additional_participants | ||
) | [inline, protected] |
Definition at line 159 of file group_session/service.h.
References _database, _reservation_count, _session_count, confirm_reservation_activation(), created(), DatabaseTransaction::end(), GroupSession, and recount_sessions().
unsigned int GroupSessionService::cancel_reservation | ( | const gsid_type | gsid, |
const uid_type | requestor, | ||
const MessageInfo & | |||
) | [protected, virtual] |
Definition at line 153 of file group_session/service.cc.
References _database, _reservation_count, and DatabaseTransaction::end().
Referenced by process_request().
unsigned int GroupSessionService::check_for_expirations | ( | const sec_type | now | ) | [virtual] |
Definition at line 128 of file group_session/service.cc.
References _database, _reservation_count, _session_count, and DatabaseTransaction::end().
Referenced by transition().
virtual void GroupSessionService::confirm_reservation_activation | ( | const GroupSession & | session | ) | [inline, protected, virtual] |
Definition at line 153 of file group_session/service.h.
References _gs_name.
Referenced by activate_reservation().
virtual void GroupSessionService::end_group_session | ( | const gsid_type | gsid | ) | [inline, protected, virtual] |
Definition at line 125 of file group_session/service.h.
References _database, _session_count, and DatabaseTransaction::end().
Referenced by process_request().
gsid_type GroupSessionService::generate_gsid | ( | ) | [inline, protected] |
Definition at line 106 of file group_session/service.h.
References _random.
Referenced by process_request().
virtual bool GroupSessionService::insert_reservation | ( | const Reservation & | reservation, |
ByteBuffer & | payload | ||
) | [inline, protected, virtual] |
Definition at line 139 of file group_session/service.h.
References _database, and _reservation_count.
Referenced by process_request().
void GroupSessionService::process_request | ( | MessageCreateGroupSession & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 184 of file group_session/service.cc.
References _database, _session_count, created(), DatabaseTransaction::end(), expires(), generate_gsid(), GroupSession, and start_group_session().
void GroupSessionService::process_request | ( | const MessageEndGroupSession & | msg, |
const MessageInfo & | |||
) | [inline, protected] |
Definition at line 133 of file group_session/service.h.
References end_group_session().
void GroupSessionService::process_request | ( | MessageCreateReservation & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 222 of file group_session/service.cc.
References _database, created(), DatabaseTransaction::end(), generate_gsid(), insert_reservation(), and recount_sessions().
void GroupSessionService::process_request | ( | const MessageFindGroupSessionsByType & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 255 of file group_session/service.cc.
References _database, and WISP_IMPORT().
void GroupSessionService::process_request | ( | const MessageFindGroupSessions & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 278 of file group_session/service.cc.
References _database, _gs_name, and _session_count.
void GroupSessionService::process_request | ( | const MessageFindMembers & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 298 of file group_session/service.cc.
References _database.
void GroupSessionService::process_request | ( | const MessageAddMembers & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 318 of file group_session/service.cc.
References _database, and DatabaseTransaction::end().
void GroupSessionService::process_request | ( | const MessageRemoveMembers & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 337 of file group_session/service.cc.
References _database, and DatabaseTransaction::end().
void GroupSessionService::process_request | ( | const MessageAddMember & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 356 of file group_session/service.cc.
References _database, _gs_name, GroupSessionProtocol::AddMemberDuplicateEntry, GroupSessionProtocol::AddMemberInternalError, GroupSessionProtocol::AddMemberMaxObserversReached, GroupSessionProtocol::AddMemberNonexistentGroupSession, GroupSessionProtocol::AddMemberSuccess, max_observers(), and GroupSessionProtocol::Observer.
void GroupSessionService::process_request | ( | const MessageRemoveMember & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 401 of file group_session/service.cc.
References _database, _gs_name, GroupSessionProtocol::Observer, and GroupSessionProtocol::Participant.
void GroupSessionService::process_request | ( | const MessageCancelReservation & | msg, |
const MessageInfo & | msginfo | ||
) | [inline, protected] |
Definition at line 226 of file group_session/service.h.
References cancel_reservation().
void GroupSessionService::process_request | ( | const MessageFindGroupSessionsForMember & | msg, |
const MessageInfo & | msginfo | ||
) | [protected] |
Definition at line 432 of file group_session/service.cc.
void GroupSessionService::process_request | ( | const MessageRelayEvent & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 450 of file group_session/service.cc.
References _database.
void GroupSessionService::process_request | ( | const MessageRelayEventSelfDiscard & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 467 of file group_session/service.cc.
References _database.
void GroupSessionService::process_request | ( | MessageRelayEvents & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 483 of file group_session/service.cc.
References _database.
void GroupSessionService::process_request | ( | MessageRelayEventsSelfDiscard & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 499 of file group_session/service.cc.
References _database.
void GroupSessionService::process_request | ( | const MessageExpireSession & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 514 of file group_session/service.cc.
References _database, DatabaseTransaction::end(), WebServiceProtocol::event_queue_ws_group(), and web_event_indirect().
void GroupSessionService::process_request | ( | const MessageLoginSession & | msg, |
const MessageInfo & | |||
) | [protected] |
Definition at line 566 of file group_session/service.cc.
References _database, WebServiceProtocol::event_queue_ws_group(), uid(), and web_event_indirect().
void GroupSessionService::recount_sessions | ( | ) | [inline, protected] |
Definition at line 110 of file group_session/service.h.
References _database, _reservation_count, and _session_count.
Referenced by activate_reservation(), and process_request().
virtual void GroupSessionService::start_group_session | ( | const GroupSession & | session, |
ByteBuffer & | payload | ||
) | [inline, protected, virtual] |
Definition at line 115 of file group_session/service.h.
References _gs_name.
Referenced by process_request().
void GroupSessionService::transition | ( | State | state | ) | [protected, virtual] |
Definition at line 40 of file group_session/service.cc.
References _gs_poll_interval, _gs_poll_timeout, _partition_id, _protocol_id, check_for_expirations(), SessionProtocol::event_group_expire(), SessionProtocol::event_group_login(), gs_protocol_group(), and gs_protocol_partition_group().
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageCreateGroupSession | |||
) | [protected] |
Referenced by process_request().
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageEndGroupSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageExpireGroupSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRelayEvent | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRelayEventSelfDiscard | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRelayEvents | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRelayEventsSelfDiscard | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindGroupSessions | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindGroupSessionsResult | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindGroupSessionsForMember | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageReturnGroupSessionsForMember | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindGroupSessionsByType | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageReturnGroupSessionsByType | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageCreateReservation | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageCancelReservation | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindMembers | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageFindMembersResult | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageAddMembers | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRemoveMembers | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageAddMember | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
MessageRemoveMember | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | SessionProtocol | , |
MessageExpireSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | SessionProtocol | , |
MessageLoginSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallStartGroupSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallExpireGroupSession | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallReturnGroupSessionsForMember | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallReturnGroupSessionsByType | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallFindGroupSessionsResult | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallFindMembersResult | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallAddMemberConfirm | |||
) | [protected] |
GroupSessionService::WISP_IMPORT | ( | protocol_traits | , |
CallRemoveMemberConfirm | |||
) | [protected] |
Friends And Related Function Documentation
friend class NS_SSRC_WISP_SERVICE::ServiceProtocolProcessor< packing_traits > [friend] |
Definition at line 51 of file group_session/service.h.
Member Data Documentation
boost::scoped_ptr<GroupSessionDatabase> GroupSessionService::_database [protected] |
Definition at line 98 of file group_session/service.h.
Referenced by activate_reservation(), cancel_reservation(), check_for_expirations(), end_group_session(), insert_reservation(), process_request(), and recount_sessions().
const std::string GroupSessionService::_gs_name [protected] |
Definition at line 104 of file group_session/service.h.
Referenced by confirm_reservation_activation(), process_request(), and start_group_session().
TimeValue GroupSessionService::_gs_poll_interval [protected] |
Definition at line 101 of file group_session/service.h.
Referenced by transition().
timeout_ptr GroupSessionService::_gs_poll_timeout [protected] |
Definition at line 102 of file group_session/service.h.
Referenced by transition().
const gsid_type GroupSessionService::_gsid_max [protected] |
Definition at line 99 of file group_session/service.h.
const gsid_type GroupSessionService::_gsid_min [protected] |
Definition at line 99 of file group_session/service.h.
const unsigned int GroupSessionService::_ids_per_expiration_message [protected] |
Definition at line 97 of file group_session/service.h.
const unsigned int GroupSessionService::_num_partitions [protected] |
Definition at line 96 of file group_session/service.h.
const unsigned int GroupSessionService::_partition_id [protected] |
Definition at line 95 of file group_session/service.h.
Referenced by transition().
const unsigned int GroupSessionService::_protocol_id [protected] |
Definition at line 94 of file group_session/service.h.
Referenced by transition().
Random<gsid_type> GroupSessionService::_random [protected] |
Definition at line 100 of file group_session/service.h.
Referenced by generate_gsid().
unsigned int GroupSessionService::_reservation_count [protected] |
Definition at line 103 of file group_session/service.h.
Referenced by activate_reservation(), cancel_reservation(), check_for_expirations(), insert_reservation(), and recount_sessions().
unsigned int GroupSessionService::_session_count [protected] |
Definition at line 103 of file group_session/service.h.
Referenced by activate_reservation(), check_for_expirations(), end_group_session(), process_request(), and recount_sessions().
const string GroupSessionService::EventGroupExpireGroupSession [static, protected] |
Definition at line 92 of file group_session/service.h.
The documentation for this class was generated from the following files: