#include <FCGIResponse.h>
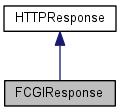
Public Member Functions | |
FCGIResponse (const boost::shared_ptr< FCGIRequest > &request) | |
virtual | ~FCGIResponse () |
void | fcgi_output (const char *content=0, const unsigned int content_length=0, bool cache_disable=CacheDisable) |
void | fcgx_finish (const HTTPStatusCode status) |
virtual void | complete (const char *content=0, const unsigned int content_length=0, bool cache_disable=CacheDisable) |
Completes the response, rendering the response to the client. | |
virtual bool | completed () const |
Returns true if the response has completed, false if not. | |
virtual void | suspend () |
Suspends the response, indicating it will be completed later via a continuation. | |
virtual void | resume () |
Resumes the response, indicating it is being continued via a continuation. | |
virtual bool | suspended () const |
Returns true if the response has been suspended for later completion, false if not. | |
virtual void | send_error (const HTTPStatusCode status) |
Sends the specified status code and renders a basic error page. | |
virtual void | send_redirect (const string &redirect) |
Redirects the client to a new URL. | |
virtual void | set_content_type (const string &type) |
Sets the content type of the response. | |
virtual const string & | content_type () const |
Returns the content type previously set by set_contetn_type(const string & type). | |
virtual void | set_status (const HTTPStatusCode status) |
Sets the status code for the response. | |
virtual HTTPStatusCode | status () const |
Returns the status code previously set by set_status(const HTTPStatus code). | |
virtual void | set_session_id (const sid_type &session_id, const unsigned int max_age=1, const bool secure=false) |
Sets the session id to store on the client. | |
virtual void | clear_session_id () |
Instructs the client to expire the current session id. | |
virtual const sid_type & | session_id () const |
Returns either the session id provided with the original request or the session id that will be sent back to the client. |
Detailed Description
Definition at line 34 of file FCGIResponse.h.
Constructor & Destructor Documentation
FCGIResponse::FCGIResponse | ( | const boost::shared_ptr< FCGIRequest > & | request | ) | [inline, explicit] |
Definition at line 48 of file FCGIResponse.h.
References SessionIdNumChars.
virtual FCGIResponse::~FCGIResponse | ( | ) | [inline, virtual] |
Definition at line 59 of file FCGIResponse.h.
References completed(), send_error(), and StatusInternalServerError.
Member Function Documentation
virtual void FCGIResponse::clear_session_id | ( | ) | [inline, virtual] |
Instructs the client to expire the current session id.
Implements HTTPResponse.
Definition at line 131 of file FCGIResponse.h.
virtual void FCGIResponse::complete | ( | const char * | content = 0 , |
const unsigned int | content_length = 0 , |
||
bool | cache_disable = CacheDisable |
||
) | [inline, virtual] |
Completes the response, rendering the response to the client.
- Parameters:
-
content A pointer to final data to send to client. content_length Length of final content to send to client. cache_disable Specifies whether or not the client should cache output. A value of CacheDisable disables caching and CacheEnable enables caching.
Implements HTTPResponse.
Definition at line 76 of file FCGIResponse.h.
References fcgi_output(), fcgx_finish(), and status().
virtual bool FCGIResponse::completed | ( | ) | const [inline, virtual] |
Returns true if the response has completed, false if not.
- Returns:
- True if the response has completed, false if not.
Implements HTTPResponse.
Definition at line 84 of file FCGIResponse.h.
Referenced by ~FCGIResponse().
virtual const string& FCGIResponse::content_type | ( | ) | const [inline, virtual] |
Returns the content type previously set by set_contetn_type(const string & type).
The initial value is text/html.
- Returns:
- The content type previously set by set_contetn_type(const string & type).
Implements HTTPResponse.
Definition at line 115 of file FCGIResponse.h.
Referenced by fcgi_output().
void FCGIResponse::fcgi_output | ( | const char * | content = 0 , |
const unsigned int | content_length = 0 , |
||
bool | cache_disable = CacheDisable |
||
) |
Definition at line 50 of file FCGIResponse.cc.
References content_type(), and status().
Referenced by complete().
void FCGIResponse::fcgx_finish | ( | const HTTPStatusCode | status | ) | [inline] |
Definition at line 69 of file FCGIResponse.h.
References set_status().
Referenced by complete(), send_error(), and send_redirect().
virtual void FCGIResponse::resume | ( | ) | [inline, virtual] |
Resumes the response, indicating it is being continued via a continuation.
Implements HTTPResponse.
Definition at line 92 of file FCGIResponse.h.
virtual void FCGIResponse::send_error | ( | const HTTPStatusCode | status | ) | [inline, virtual] |
Sends the specified status code and renders a basic error page.
- Parameters:
-
status The status code to send.
Implements HTTPResponse.
Definition at line 100 of file FCGIResponse.h.
References fcgx_finish().
Referenced by send_redirect(), and ~FCGIResponse().
void FCGIResponse::send_redirect | ( | const string & | redirect | ) | [virtual] |
Redirects the client to a new URL.
If the redirect target is not an absolute URL, it is converted to an absolute URL based on the original request URI. A relative path with no leading / will interpret the original request URI as a parent path. Therefore, if you're redirecting a request URI that does not map to a directory, you should calculate the parent path and create a path with a leading / from it.
- Parameters:
-
redirect The relative, or absolute URL of the redirect target.
Implements HTTPResponse.
Definition at line 76 of file FCGIResponse.cc.
References fcgx_finish(), send_error(), StatusFound, and StatusInternalServerError.
virtual const sid_type& FCGIResponse::session_id | ( | ) | const [inline, virtual] |
Returns either the session id provided with the original request or the session id that will be sent back to the client.
If no session id was provided by the client and no session id has been set via set_sesion_id(const string &), it returns an empty string. Otherwise it returns the session id provided by the client if set_session_id has not been called, else the id set via set_session_id.
- Returns:
- The sesion id provided with the original request or set via set_session_id.
Implements HTTPResponse.
Definition at line 144 of file FCGIResponse.h.
Referenced by set_session_id().
virtual void FCGIResponse::set_content_type | ( | const string & | type | ) | [inline, virtual] |
Sets the content type of the response.
In general, this method should be used only for responses that aren't text/html.
- Parameters:
-
type The content type to set.
Implements HTTPResponse.
Definition at line 111 of file FCGIResponse.h.
__BEGIN_NS_SSRC_WSPR_FCGI void FCGIResponse::set_session_id | ( | const sid_type & | session_id, |
const unsigned int | max_age = 1 , |
||
const bool | secure = false |
||
) | [virtual] |
Sets the session id to store on the client.
- Parameters:
-
session_id The new session id to set. max_age The lifetime of the cookie in seconds. A value of 1 is interpreted specially to mean the cookie expires when the user agent terminates.
Implements HTTPResponse.
Definition at line 26 of file FCGIResponse.cc.
References session_id().
virtual void FCGIResponse::set_status | ( | const HTTPStatusCode | status | ) | [inline, virtual] |
Sets the status code for the response.
In general, this method should be used only for responses that don't constitute errors. To render an error page along with an error status, you should use send_error(const HTTPStatusCode).
- Parameters:
-
status The status code to set.
Implements HTTPResponse.
Definition at line 119 of file FCGIResponse.h.
References status().
Referenced by fcgx_finish().
virtual HTTPStatusCode FCGIResponse::status | ( | ) | const [inline, virtual] |
Returns the status code previously set by set_status(const HTTPStatus code).
The initial value is StatusOK.
- Returns:
- The status code previously set by set_status(const HTTPStatus code).
Implements HTTPResponse.
Definition at line 123 of file FCGIResponse.h.
Referenced by complete(), fcgi_output(), and set_status().
virtual void FCGIResponse::suspend | ( | ) | [inline, virtual] |
Suspends the response, indicating it will be completed later via a continuation.
Implements HTTPResponse.
Definition at line 88 of file FCGIResponse.h.
virtual bool FCGIResponse::suspended | ( | ) | const [inline, virtual] |
Returns true if the response has been suspended for later completion, false if not.
- Returns:
- True if the response has been suspended for later completion, false if not.
Implements HTTPResponse.
Definition at line 96 of file FCGIResponse.h.
The documentation for this class was generated from the following files: