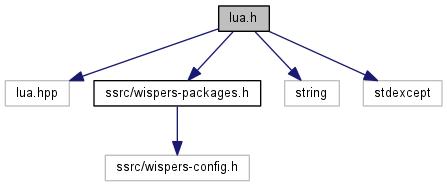
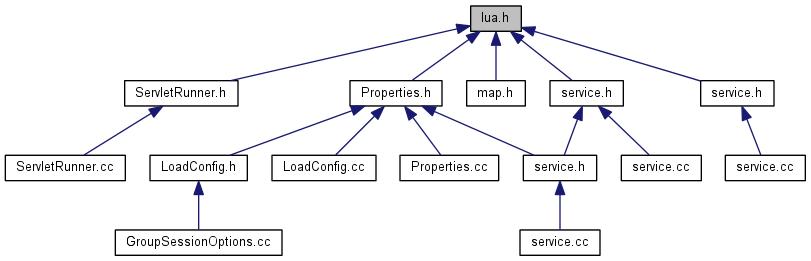
Go to the source code of this file.
Classes | |
struct | LuaCallError |
Namespaces | |
namespace | Lua |
The Lua C API from <lua.hpp> can be accessed via the Lua namespace if required, but it is intended for internal use by the library to avoid polluting the global namespace. | |
namespace | detail |
Functions | |
template<typename R > | |
R | convert (lua_State *state, int index=-1) |
template<> | |
bool | convert< bool > (lua_State *state, int index) |
template<> | |
int | convert< int > (lua_State *state, int index) |
template<> | |
unsigned int | convert< unsigned int > (lua_State *state, int index) |
template<> | |
string | convert< string > (lua_State *state, int index) |
template<> | |
const char * | convert< const char * > (lua_State *state, int index) |
template<> | |
lua_Number | convert< lua_Number > (lua_State *state, int index) |
void | push_value (lua_State *state, const bool value) |
void | push_value (lua_State *state, const int value) |
void | push_value (lua_State *state, const lua_Number value) |
void | push_value (lua_State *state, const char *value) |
void | push_value (lua_State *state, const string &value) |
void | push_value (lua_State *state, void *value) |
void | push_value (lua_State *state, const void *value) |
void | push_values (lua_State *) |
template<typename P > | |
void | push_values (lua_State *state, const P &p) |
template<typename T , typename... P> | |
void | push_values (lua_State *state, const T &t, const P &...p) |
template<typename V > | |
void | set_field (lua_State *state, int index, const V &value, const char *const field) |
template<typename V > | |
void | set_field (lua_State *state, int index, const V &value, const string &field) |
template<typename V > | |
void | set_field (lua_State *state, int index, const V &value, int n) |
void | detail::_get_field (lua_State *state, int index, const char *const field) |
void | detail::_get_field (lua_State *state, int index, const string &field) |
template<typename V > | |
void | detail::_create_value (lua_State *state, const V &value, const char *const field) |
template<typename V > | |
void | detail::_create_value (lua_State *state, const V &value, const string &field) |
template<typename V , typename... P> | |
void | detail::_create_value (lua_State *state, const V &value, const char *const field, P...p) |
template<typename V > | |
void | detail::_set_value (lua_State *state, const V &value, const char *const field) |
template<typename V > | |
void | detail::_set_value (lua_State *state, const V &value, const string &field) |
template<typename V , typename... P> | |
void | detail::_set_value (lua_State *state, const V &value, const char *const field, P...p) |
void | get_global (lua_State *state, const char *const field) |
void | get_global (lua_State *state, const string &field) |
template<typename V > | |
void | set_global (lua_State *state, const V &value, const char *const field) |
template<typename V > | |
void | set_global (lua_State *state, const V &value, const string &field) |
template<typename R > | |
const R | get_field (const R &default_value, lua_State *state, int index, const char *const field) |
template<typename R > | |
const R | get_field (const R &default_value, lua_State *state, int index, const string &field) |
template<typename R > | |
const R | get_field (const R &default_value, lua_State *state, int index, int n) |
template<typename R , typename... P> | |
const R | get_field (const R &default_value, lua_State *state, int index, const char *const field, P...p) |
template<typename R > | |
const R | get_field (lua_State *state, int index, const char *const field) |
template<typename R > | |
const R | get_field (lua_State *state, int index, const string &field) |
template<typename R > | |
const R | get_field (lua_State *state, int index, int n) |
template<typename R , typename... P> | |
const R | get_field (lua_State *state, int index, const char *const field, P...p) |
template<typename R > | |
const R | get_value (const R &default_value, lua_State *state, const char *const var) |
template<typename R > | |
const R | get_value (const R &default_value, lua_State *state, const string &var) |
template<typename R , typename... P> | |
const R | get_value (const R &default_value, lua_State *state, const char *const var, P...p) |
template<typename R > | |
const R | get_value (lua_State *state, const char *const var) |
template<typename R > | |
const R | get_value (lua_State *state, const string &var) |
template<typename R , typename... P> | |
const R | get_value (lua_State *state, const char *const var, P...p) |
template<typename V > | |
void | create_value (lua_State *state, const V &value, const char *const var) |
template<typename V > | |
void | create_value (lua_State *state, const V &value, const string &var) |
template<typename V , typename... P> | |
void | create_value (lua_State *state, const V &value, const char *const var, P...p) |
template<typename V , typename... P> | |
void | set_field (lua_State *state, int index, const V &value, const char *const field, P...p) |
template<typename V > | |
void | set_value (lua_State *state, const V &value, const char *const var) |
template<typename V > | |
void | set_value (lua_State *state, const V &value, const string &var) |
template<typename V , typename... P> | |
void | set_value (lua_State *state, const V &value, const char *const var, P...p) |
template<typename I > | |
void | prepend_package_path (lua_State *state, const I &begin, const I &end) |
template<typename... P> | |
void | pcall_nopop (const int nargs, const int nresults, lua_State *state, P...p) SSRC_DECL_THROW(LuaCallError) |
Assumes function has already been pushed to top of stack. | |
template<typename... P> | |
void | pcall_nopop (const int nresults, lua_State *state, P...p) SSRC_DECL_THROW(LuaCallError) |
template<typename R , typename... P> | |
R | pcall_ret (lua_State *state, const P &...p) SSRC_DECL_THROW(LuaCallError) |
Currently, we support only one return value, but it should be possible to work things out to specify multiple return values and return them via a tuple. | |
template<typename... P> | |
void | pcall (lua_State *state, const P &...p) SSRC_DECL_THROW(LuaCallError) |
Function Documentation
R convert | ( | lua_State * | state, |
int | index = -1 |
||
) | [inline] |
bool convert< bool > | ( | lua_State * | state, |
int | index | ||
) | [inline] |
Definition at line 46 of file lua.h.
Referenced by table_to_properties().
const char* convert< const char * > | ( | lua_State * | state, |
int | index | ||
) | [inline] |
int convert< int > | ( | lua_State * | state, |
int | index | ||
) | [inline] |
lua_Number convert< lua_Number > | ( | lua_State * | state, |
int | index | ||
) | [inline] |
Definition at line 69 of file lua.h.
Referenced by table_to_properties().
Definition at line 59 of file lua.h.
Referenced by table_to_properties().
unsigned int convert< unsigned int > | ( | lua_State * | state, |
int | index | ||
) | [inline] |
void create_value | ( | lua_State * | state, |
const V & | value, | ||
const char *const | var | ||
) | [inline] |
Definition at line 352 of file lua.h.
References set_global().
void create_value | ( | lua_State * | state, |
const V & | value, | ||
const string & | var | ||
) | [inline] |
Definition at line 359 of file lua.h.
References set_global().
void create_value | ( | lua_State * | state, |
const V & | value, | ||
const char *const | var, | ||
P... | p | ||
) | [inline] |
Definition at line 366 of file lua.h.
References detail::_create_value().
const R get_field | ( | const R & | default_value, |
lua_State * | state, | ||
int | index, | ||
const char *const | field | ||
) | [inline] |
Definition at line 222 of file lua.h.
Referenced by get_field().
const R get_field | ( | const R & | default_value, |
lua_State * | state, | ||
int | index, | ||
const string & | field | ||
) | [inline] |
Definition at line 235 of file lua.h.
References detail::_get_field().
const R get_field | ( | const R & | default_value, |
lua_State * | state, | ||
int | index, | ||
int | n | ||
) | [inline] |
const R get_field | ( | const R & | default_value, |
lua_State * | state, | ||
int | index, | ||
const char *const | field, | ||
P... | p | ||
) | [inline] |
const R get_field | ( | lua_State * | state, |
int | index, | ||
const char *const | field | ||
) | [inline] |
Definition at line 276 of file lua.h.
References get_field().
const R get_field | ( | lua_State * | state, |
int | index, | ||
const string & | field | ||
) | [inline] |
Definition at line 281 of file lua.h.
References get_field().
const R get_field | ( | lua_State * | state, |
int | index, | ||
int | n | ||
) | [inline] |
Definition at line 286 of file lua.h.
References get_field().
const R get_field | ( | lua_State * | state, |
int | index, | ||
const char *const | field, | ||
P... | p | ||
) | [inline] |
Definition at line 291 of file lua.h.
References get_field().
void get_global | ( | lua_State * | state, |
const char *const | field | ||
) | [inline] |
Definition at line 196 of file lua.h.
Referenced by get_value(), prepend_package_path(), and set_value().
void get_global | ( | lua_State * | state, |
const string & | field | ||
) | [inline] |
Definition at line 200 of file lua.h.
References push_value().
const R get_value | ( | const R & | default_value, |
lua_State * | state, | ||
const char *const | var | ||
) | [inline] |
const R get_value | ( | const R & | default_value, |
lua_State * | state, | ||
const string & | var | ||
) | [inline] |
Definition at line 312 of file lua.h.
References get_global().
const R get_value | ( | const R & | default_value, |
lua_State * | state, | ||
const char *const | var, | ||
P... | p | ||
) | [inline] |
Definition at line 324 of file lua.h.
References get_global().
const R get_value | ( | lua_State * | state, |
const char *const | var | ||
) | [inline] |
Definition at line 337 of file lua.h.
References get_value().
Definition at line 342 of file lua.h.
References get_value().
const R get_value | ( | lua_State * | state, |
const char *const | var, | ||
P... | p | ||
) | [inline] |
Definition at line 347 of file lua.h.
References get_value().
void pcall | ( | lua_State * | state, |
const P &... | p | ||
) | [inline] |
Definition at line 478 of file lua.h.
References pcall_nopop().
Referenced by Renderer::clear_template_cache(), Relay::finish_post(), Renderer::render_and_cache(), Renderer::uncache_environment(), and Renderer::uncache_template().
void pcall_nopop | ( | const int | nargs, |
const int | nresults, | ||
lua_State * | state, | ||
P... | p | ||
) | [inline] |
Assumes function has already been pushed to top of stack.
The nargs parameter is to allow you to pre-push values onto the stack.
Definition at line 441 of file lua.h.
References push_values().
Referenced by Relay::finish_post(), Renderer::load_environment(), pcall(), pcall_nopop(), pcall_ret(), ServletRunner::process_fcgi_request(), Renderer::render_and_cache(), and Renderer::send_error().
void pcall_nopop | ( | const int | nresults, |
lua_State * | state, | ||
P... | p | ||
) | [inline] |
Definition at line 456 of file lua.h.
References pcall_nopop().
R pcall_ret | ( | lua_State * | state, |
const P &... | p | ||
) | [inline] |
Currently, we support only one return value, but it should be possible to work things out to specify multiple return values and return them via a tuple.
Definition at line 468 of file lua.h.
References pcall_nopop().
void prepend_package_path | ( | lua_State * | state, |
const I & | begin, | ||
const I & | end | ||
) | [inline] |
Definition at line 412 of file lua.h.
References get_global(), and set_field().
Referenced by _lua_init_global_environment_ref(), and ServletRunner::ServletRunner().
void push_value | ( | lua_State * | state, |
const bool | value | ||
) | [inline] |
Definition at line 73 of file lua.h.
Referenced by detail::_get_field(), Relay::finish_post(), get_global(), map_to_table(), push_values(), set_field(), and set_global().
void push_value | ( | lua_State * | state, |
const int | value | ||
) | [inline] |
void push_value | ( | lua_State * | state, |
const lua_Number | value | ||
) | [inline] |
void push_value | ( | lua_State * | state, |
const char * | value | ||
) | [inline] |
void push_value | ( | lua_State * | state, |
const string & | value | ||
) | [inline] |
void push_value | ( | lua_State * | state, |
void * | value | ||
) | [inline] |
void push_value | ( | lua_State * | state, |
const void * | value | ||
) | [inline] |
void push_values | ( | lua_State * | ) | [inline] |
Definition at line 102 of file lua.h.
Referenced by pcall_nopop(), and push_values().
void push_values | ( | lua_State * | state, |
const P & | p | ||
) | [inline] |
Definition at line 105 of file lua.h.
References push_value().
void push_values | ( | lua_State * | state, |
const T & | t, | ||
const P &... | p | ||
) | [inline] |
Definition at line 110 of file lua.h.
References push_value(), and push_values().
void set_field | ( | lua_State * | state, |
int | index, | ||
const V & | value, | ||
const char *const | field | ||
) | [inline] |
Definition at line 116 of file lua.h.
References push_value().
Referenced by detail::_create_value(), detail::_set_value(), map_to_table(), prepend_package_path(), and set_field().
void set_field | ( | lua_State * | state, |
int | index, | ||
const V & | value, | ||
const string & | field | ||
) | [inline] |
Definition at line 124 of file lua.h.
References push_value().
void set_field | ( | lua_State * | state, |
int | index, | ||
const V & | value, | ||
int | n | ||
) | [inline] |
Definition at line 133 of file lua.h.
References push_value().
void set_field | ( | lua_State * | state, |
int | index, | ||
const V & | value, | ||
const char *const | field, | ||
P... | p | ||
) | [inline] |
Definition at line 375 of file lua.h.
References set_field().
void set_global | ( | lua_State * | state, |
const V & | value, | ||
const char *const | field | ||
) | [inline] |
Definition at line 206 of file lua.h.
References push_value().
Referenced by create_value(), and set_value().
void set_global | ( | lua_State * | state, |
const V & | value, | ||
const string & | field | ||
) | [inline] |
Definition at line 214 of file lua.h.
References push_value().
void set_value | ( | lua_State * | state, |
const V & | value, | ||
const char *const | var | ||
) | [inline] |
Definition at line 390 of file lua.h.
References set_global().
Referenced by Relay::finish_post(), and Renderer::render_and_cache().
void set_value | ( | lua_State * | state, |
const V & | value, | ||
const string & | var | ||
) | [inline] |
Definition at line 397 of file lua.h.
References set_global().
void set_value | ( | lua_State * | state, |
const V & | value, | ||
const char *const | var, | ||
P... | p | ||
) | [inline] |
Definition at line 402 of file lua.h.
References detail::_set_value(), and get_global().