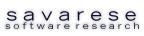
Go to the documentation of this file.
21 #ifndef __SSRC_WISP_UTILITY_WISP_STRUCT_H
22 #define __SSRC_WISP_UTILITY_WISP_STRUCT_H
24 #include <boost/preprocessor/punctuation/comma_if.hpp>
25 #include <boost/preprocessor/control/expr_iif.hpp>
26 #include <boost/preprocessor/comparison/equal.hpp>
27 #include <boost/preprocessor/comparison/less_equal.hpp>
28 #include <boost/preprocessor/comparison/greater_equal.hpp>
29 #include <boost/preprocessor/seq.hpp>
30 #include <boost/preprocessor/array/elem.hpp>
31 #include <boost/preprocessor/array/push_front.hpp>
32 #include <boost/preprocessor/stringize.hpp>
33 #include <boost/call_traits.hpp>
43 template<
class Archive>
void serialize(Archive & ar,
const unsigned int) { }
48 #define __WISP_STRUCT_MEMBER(r, data, arg) \
49 BOOST_PP_TUPLE_ELEM(2, 0, arg) BOOST_PP_TUPLE_ELEM(2, 1, arg);
51 #define __WISP_STRUCT_DEFAULT_INIT_MEMBER(r, data, i, arg) \
52 BOOST_PP_COMMA_IF(i) BOOST_PP_TUPLE_ELEM(2, 1, arg)()
54 #define __WISP_STRUCT_INIT_MEMBER(r, data, i, arg) \
55 BOOST_PP_COMMA_IF(i) BOOST_PP_TUPLE_ELEM(2, 1, arg)(BOOST_PP_TUPLE_ELEM(2, 1, arg))
57 #define __WISP_STRUCT_PARAM(r, in_template, i, arg) \
58 BOOST_PP_COMMA_IF(i) BOOST_PP_EXPR_IIF(in_template, typename) boost::call_traits<BOOST_PP_TUPLE_ELEM(2, 0, arg)>::param_type BOOST_PP_TUPLE_ELEM(2, 1, arg)
60 #define __WISP_STRUCT_PARAM_INIT(r, init, i, arg) \
61 BOOST_PP_COMMA_IF(i) BOOST_PP_EXPR_IIF(BOOST_PP_ARRAY_ELEM(0, init), typename) boost::call_traits<BOOST_PP_TUPLE_ELEM(2, 0, arg)>::param_type BOOST_PP_TUPLE_ELEM(2, 1, arg) BOOST_PP_EXPR_IIF(BOOST_PP_GREATER_EQUAL(i, BOOST_PP_ARRAY_ELEM(1, init)), = BOOST_PP_ARRAY_ELEM(BOOST_PP_SUB(BOOST_PP_ADD(i, 2), BOOST_PP_ARRAY_ELEM(1, init)), init))
63 #define __WISP_STRUCT_SERIALIZE(r, data, arg) & BOOST_PP_TUPLE_ELEM(2, 1, arg)
66 #define __WISP_STRUCT_SERIALIZE_MEMBERS(members) \
67 template<class Archive> \
68 void serialize(Archive & ar, const unsigned int) { \
69 ar BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_SERIALIZE, _, members); \
73 #define __WISP_STRUCT_EQOP_TEST(r, data, i, arg) \
74 BOOST_PP_EXPR_IF(i, &&) (o1.BOOST_PP_TUPLE_ELEM(2, 1, arg) == o2.BOOST_PP_TUPLE_ELEM(2, 1, arg))
76 #define __WISP_STRUCT_EQOP(name, seq) \
77 friend bool operator==(const name & o1, const name & o2) { \
78 return (BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_EQOP_TEST, _, seq)); \
88 #define __WISP_STRUCT_VISIT_MEMBERS(r, v, arg) \
89 (BOOST_PP_STRINGIZE(BOOST_PP_TUPLE_ELEM(2, 1, arg)), BOOST_PP_TUPLE_ELEM(2, 1, arg))
93 #define __WISP_STRUCT_VISIT(members) \
94 template<typename Visitor> \
95 void visit(Visitor && visitor) { \
96 visitor BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_VISIT_MEMBERS, visitor, members); \
98 template<typename Visitor> \
99 void visit(Visitor && visitor) const { \
100 visitor BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_VISIT_MEMBERS, visitor, members); \
103 #define __WISP_STRUCT_VISIT(members)
109 #define __WISP_STRUCT(in_template, name, members) \
110 BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_MEMBER, _, members) \
112 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_DEFAULT_INIT_MEMBER, _, members) { } \
113 explicit name(BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_PARAM, in_template, members)) : \
114 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_INIT_MEMBER, _, members) { } \
115 __WISP_STRUCT_SERIALIZE_MEMBERS(members) \
116 __WISP_STRUCT_EQOP(name, members) \
117 __WISP_STRUCT_VISIT(members)
119 #define __WISP_STRUCT_WITH_INIT(in_template, name, members, initializers) \
120 BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_MEMBER, _, members) \
121 explicit name(BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_PARAM_INIT, BOOST_PP_ARRAY_PUSH_FRONT(BOOST_PP_ARRAY_PUSH_FRONT(initializers, BOOST_PP_SUB(BOOST_PP_SEQ_SIZE(members), BOOST_PP_ARRAY_SIZE(initializers))), in_template), members)) : \
122 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_INIT_MEMBER, _, members) { } \
123 __WISP_STRUCT_SERIALIZE_MEMBERS(members) \
124 __WISP_STRUCT_EQOP(name, members) \
125 __WISP_STRUCT_VISIT(members)
127 #define WISP_STRUCT(name, members) \
128 struct name : public NS_SSRC_WISP_UTILITY::wisp_struct { __WISP_STRUCT(0, name, members) }
130 #define WISP_STRUCT_T(name, members) \
131 struct name : public NS_SSRC_WISP_UTILITY::wisp_struct { __WISP_STRUCT(1, name, members) }
133 #define WISP_STRUCT_WITH_INIT(name, members, initializers) \
134 struct name : public NS_SSRC_WISP_UTILITY::wisp_struct { __WISP_STRUCT_WITH_INIT(0, name, members, BOOST_PP_SEQ_TO_ARRAY(initializers)) }
136 #define WISP_STRUCT_WITH_INIT_T(name, members, initializers) \
137 struct name : public NS_SSRC_WISP_UTILITY::wisp_struct { __WISP_STRUCT_WITH_INIT(1, name, members, BOOST_PP_SEQ_TO_ARRAY(initializers)) }
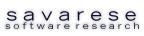
Copyright © 2006-2012 Savarese Software Research Corporation. All rights reserved.