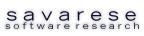
Go to the documentation of this file.
22 #ifndef __SSRC_WISP_PROTOCOL_SERVICE_PROTOCOL_H
23 #define __SSRC_WISP_PROTOCOL_SERVICE_PROTOCOL_H
29 #include <ssrc/spread/Message.h>
45 template<wisp_message_protocol protocol_,
typename PT = BinaryPackingTraits>
53 #define WISP_DEFINE_PROTOCOL(protocol_base) \
54 template<NS_SSRC_WISP_PROTOCOL::wisp_message_id _id> \
55 struct Message ## protocol_base : public NS_SSRC_WISP_UTILITY::wisp_struct { \
56 enum { protocol = protocol_base }; \
58 template<class Archive> \
59 void serialize(Archive & ar, const unsigned int) { } \
64 #define __WISP_PROTOCOL_MESSAGE(in_template, call, base, members) \
65 struct BOOST_PP_CAT(Message, call) : public base<call> { \
66 BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_MEMBER, _, members) \
67 BOOST_PP_CAT(Message, call)() : \
68 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_DEFAULT_INIT_MEMBER, _, members) { } \
69 explicit BOOST_PP_CAT(Message, call)(BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_PARAM, in_template, members)) : \
70 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_INIT_MEMBER, _, members) { } \
71 __WISP_STRUCT_SERIALIZE_MEMBERS(members) \
72 __WISP_STRUCT_VISIT(members) \
75 #define __WISP_PROTOCOL_MESSAGE_WITH_INIT(in_template, call, base, members, initializers) \
76 struct BOOST_PP_CAT(Message, call) : public base<call> { \
77 BOOST_PP_SEQ_FOR_EACH(__WISP_STRUCT_MEMBER, _, members) \
78 explicit BOOST_PP_CAT(Message, call)(BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_PARAM_INIT, BOOST_PP_ARRAY_PUSH_FRONT(BOOST_PP_ARRAY_PUSH_FRONT(initializers, BOOST_PP_SUB(BOOST_PP_SEQ_SIZE(members), BOOST_PP_ARRAY_SIZE(initializers))), in_template), members)) : \
79 BOOST_PP_SEQ_FOR_EACH_I(__WISP_STRUCT_INIT_MEMBER, _, members) { } \
80 __WISP_STRUCT_SERIALIZE_MEMBERS(members) \
81 __WISP_STRUCT_VISIT(members) \
84 #define WISP_PROTOCOL_MESSAGE(call, base, members) \
85 __WISP_PROTOCOL_MESSAGE(0, call, base, members)
87 #define WISP_PROTOCOL_MESSAGE_T(call, base, members) \
88 __WISP_PROTOCOL_MESSAGE(1, call, base, members)
90 #define WISP_PROTOCOL_MESSAGE_WITH_INIT(call, base, members, initializers) \
91 __WISP_PROTOCOL_MESSAGE_WITH_INIT(0, call, base, members, BOOST_PP_SEQ_TO_ARRAY(initializers))
93 #define WISP_PROTOCOL_MESSAGE_WITH_INIT_T(call, base, members, initializers) \
94 __WISP_PROTOCOL_MESSAGE_WITH_INIT(1, call, base, members, BOOST_PP_SEQ_TO_ARRAY(initializers))
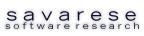
Copyright © 2006-2012 Savarese Software Research Corporation. All rights reserved.
Copyright © 2017 Savarese Software Research Corporation. All rights reserved