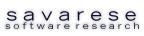
Go to the documentation of this file.
21 #ifndef __SSRC_WISP_UTILITY_BASIC_SERVICE_OPTIONS_H
22 #define __SSRC_WISP_UTILITY_BASIC_SERVICE_OPTIONS_H
26 #include <boost/program_options.hpp>
37 boost::program_options::options_description basic_description;
48 using namespace boost::program_options;
50 basic_description.add_options()
51 (
"help,h",
"Display this help message and exit.")
52 (
"connection,c", value<std::string>(&
connection),
53 "Specify the name of the Spread daemon to connect to.")
54 (
"name,n", value<std::string>(&
name),
55 "The Spread private name to assign the service.")
58 "The ssrc::spread::Message capacity the service requires for message "
59 "sends. This value should equal the size in bytes of the largest "
60 "message the service will ever send.")
63 "The number of seconds to wait before cancelling an asynchronous call "
64 "that has not yet returned.")
65 (
"locale,l", value<std::string>(&
locale),
66 "The locale to use for ctype string interpretation.");
71 virtual void store(
int argc,
char *argv[]) {
72 namespace po = boost::program_options;
74 po::store(po::parse_command_line(argc, argv,
description), vm);
79 virtual void notify(boost::program_options::variables_map & vm) {
80 namespace po = boost::program_options;
81 help = (vm.count(
"help") > 0);
86 po::variables_map::iterator it = vm.begin();
87 while(it != vm.end()) {
88 if(!it->second.empty() && !it->second.defaulted())
92 help = (it == vm.end());
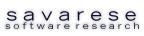
Copyright © 2006-2012 Savarese Software Research Corporation. All rights reserved.
Copyright © 2017 Savarese Software Research Corporation. All rights reserved