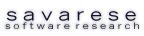
Go to the documentation of this file.
21 #ifndef __SSRC_SPREAD_GROUP_LIST_H
22 #define __SSRC_SPREAD_GROUP_LIST_H
27 #include <ssrc/spread/detail/Buffer.h>
78 return std::pair<string,string>(string(private_group, 1, length),
79 string(private_group, length + 2,
80 private_group.size() - length - 2));
96 typedef detail::Buffer<group_type> group_vector;
109 unsigned int capacity()
const {
110 return _groups.capacity();
114 _groups.resize(size);
122 explicit GroupList(
const unsigned int capacity = 10) : _groups(capacity) { }
133 gname.group[
size] = 0;
143 if(&groups !=
this) {
144 unsigned int offset =
size();
146 std::memcpy(GroupList::groups() + offset, groups.groups(),
159 string group(
const unsigned int index)
const {
160 return _groups[index].group;
175 if(&groups !=
this) {
177 std::memcpy(GroupList::groups(), groups.groups(),
193 return _groups.size();
213 unsigned int size = groups1.
size();
215 if(size != groups2.
size())
219 if(std::strncmp(groups1.groups()[
size], groups2.groups()[
size],
237 for(
unsigned int i = 0; i < groups.
size(); ++i)
238 output << groups._groups[i].group << std::endl;
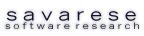
Copyright © 2006-2015 Savarese Software Research Corporation. All rights reserved.
Copyright © 2017 Savarese Software Research Corporation. All rights reserved